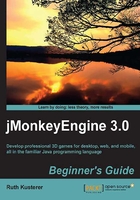
Time for action – configuring display settings
In order to control the display settings yourself, you need to create an AppSettings
object in your application's main()
method. Set the Boolean in the AppSettings
constructor to true, if you want to keep the default settings for every value that you do not specify. You can set this parameter to false if you want to specify each property yourself. The engine will then raise an exception if you miss a setting.
public static void main(String[] args) { settings.setTitle("My Cool Game"); // specify your settings here Main app = new Main(); app.setSettings(settings); // apply settings to app app.start(); // use settings and run }
The following application settings are available:
- Dialog image: If you choose to show the default display settings dialog, the window includes a splash screen. By default, the dialog box shows the jMonkeyEngine logo from the built-in
com/jme3/app/Monkey.png
. To reassure your users that this dialog box is already part of your game, you should specify a branded image from yourassets/interface
folder:settings.setSettingsDialogImage("Interface/splashscreen.png")
- Fullscreen mode: By default, the application runs in a window of its own. Alternatively, you can switch to fullscreen mode and make the application window fill the whole screen. If you activate this mode, users will not be able to move the window or access any menus. By default, pressing the Esc key stops the application and helps exit the fullscreen mode gracefully.
GraphicsDevice device = GraphicsEnvironment. getLocalGraphicsEnvironment().getDefaultScreenDevice(); DisplayMode[] modes = device.getDisplayModes(); settings.setResolution(modes[0].getWidth(),modes[0].getHeight()); settings.setFrequency(modes[0].getRefreshRate()); settings.setDepthBits(modes[0].getBitDepth()); settings.setFullscreen(device.isFullScreenSupported());
- Resolution: The default resolution of a jMonkeyEngine window is 640 × 480 pixels. There are two equivalent ways to set the display resolution. You can set the width and height separately:
settings.setHeight(480); settings.setWidth(640);
Or both resolution values in one step:
settings.setResolution(640,480);
- Anti-aliasing: If you want your scene rendered more smoothly, you can choose to activate anti-aliasing. Depending on the graphics card, the user can set the anti-aliasing feature to use 2, 4, 8, 16, or 32 samples. With anti-aliasing activated, the scenes will render with softer edges, but the game may run slower on older hardware.
settings.setSamples(2);
To switch off anti-aliasing, leave multisampling at its default value
0
. The scenes will render with harder edges, but the game may run faster. - Input handling: You can choose whether the running application listens to user input, such as a mouse and keyboard. Input handling is active (true) by default. You can deactivate input handling for use cases, where you only play a 3D scene without any user interaction. You would do that, for example, when playing a non-interactive simulation.
settings.useInput(false);
- Window title: The window title of a jMonkeyEngine application is set to
jMonkey Engine 3.0
, by default. This is where you display your game's name. The window title is not visible when the game runs in fullscreen mode.settings.setTitle("My Cool Game").
- Saving settings: A user-friendly application will ask you to specify display settings only once, and will then load them every time without asking again. In a jMonkeyEngine game, you load and save
AppSettings
objects using standardjava.io
serialization.settings.save("MyGame.prefs"); settings.load("MyGame.prefs");
Typically, you use save()
and load()
methods together with a try-catch
statement. You can copy an AppSettings
object from oldSettings
into newSettings
using the following command:
newSettings.copyFrom(oldSettings)
What just happened?
Specify the settings and apply them to the application before calling the app.start()
method in the main()
method. If you change the settings while the application is already running, use the app.restart();
method instead, to load the new AppSettings
object!
As you see, you can either specify the display settings yourself, or let users set their own options:
- Use the previous methods to specify the display settings yourself, in the
main()
method. Typically, you would create your own user interface to let users modify the settings on a custom options screen. In these cases, you should disable the default settings window at startup:app.setShowSettings(false);
- If you expect users with widely different hardware, you can let them choose the rendering options, such as the resolution, anti-aliasing, and color depth, to their liking. In this case, you can configure your application to open a Display Settings dialog box at start-up:
app.setShowSettings(true);
- Add this setter after the creation of the
app
object, but before calling theapp.start()
method.