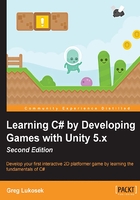
Making decisions in code
The fundamental mechanism of programming is making decisions. In everyday life, we make hundreds—and possibly thousands—of decisions a day. They might be the results of simple questions such as, "Do I need an umbrella today?" or "Should I drive at the maximum motorway speed at the moment?" Let's first take a question and draw a single graph, as follows:

This is a fairly easy question. If it will be raining, I need an umbrella; otherwise, I don't. In programming, we call it an if
statement. It's a way we describe to the computer what code should be executed under what conditions. The question "Will it be raining?" is the condition. When planning your code, you should always break down decision-making in to simple questions that can be answered only by a "yes" or a "no."
Note
In C# syntax, we use true
or false
instead of yes/no.
We now know how the simplest if
statements work. Let's see how this question will look in code. Let's create a new script, name it LearningStatements
, and add it to a GameObject
in the scene:

Look at the code on line 10 and its description:
if (willItBeRainingToday)
An if
statement is used to test whether the condition between the parentheses is true or false. The willItBeRainingToday
variable stores a value true
. Therefore, the code block in line 11 will be executed. Go ahead and hit Play in the Editor tab. The Console will print out line 11.
Line 12 contains the else
keyword. Everything within the braces after the else
keyword is executed only if the previous conditions aren't met. To test how it works, we press Stop in the editor, and on the GameObject
containing our LearningStatements
script, we change our variable value by ticking the checkbox in the Inspector panel. Then press Play again.
Using the NOT operator to change the condition
Here's a little curveball to wrap your mind around—the NOT logical operator. It's written in code using an exclamation mark. This makes a true condition false, or a false condition true. Let's add a NOT operator to our statement. Line 10 should now look like this:
if ( ! willItBeRainingToday ) {
Press Play in the editor. You will notice that the decision-making is now working the opposite way. Line 11 will be executed only if the willItBeRaining
variable is false.
Checking many conditions in an if statement
Sometimes, you will want your if
statements to check many conditions before any code block is executed. This is very easy to do. There are two more logical operators that you can use:
- AND: This is used by putting
&&
between the conditions being checked. The code inside the curly braces is executed only if all the conditions are true: - OR: This is used by putting
||
between the conditions being checked. Then, the code inside the curly braces is executed if any of the conditions are true:
Using else if to make complex decisions
So far, we have learned how to decide what code we want to execute if certain conditions are met. Using if
and else
, we can decide what code is executed out of two parts. You are probably wondering, "What if I have many more complex decisions to make and need to be able to choose between more than two code blocks?" Yes, good question!
The else if
expression is an expression that you can add after the code block belonging to the first if
statement. Don't worry; it's not complicated. Let's take another example. Imagine you are driving a car and you need to check the speed limit and decide what speed you want to drive at.

Let's analyze the code:
- Line 9: This line declares the
speedLimit
number variable and assigns a value of60
. - Line 11: The
if
statement checks whether thespeedLimit
variable is exactly70
. As we have assignedspeedLimit
as60
, the statement in line 11 is false, so line 12 won't be executed. - Line 14: The compiler will check this statement whenever the statement directly before
else
is false. Don't panic; it sounds very confusing now. All you need to know at the moment is that theelse if
statement is checked only if the previous statement isn't true. - Line 17: Analogically, line 17 is checked only if line 14 is false.
Note
Of course, you can nest if
statements inside each other. The syntax would look exactly the same. Simply write your new child if
statement between the curly braces of the parent statement.
Making decisions based on user input
Decisions always have to be made when the user provides input. Previously in this chapter, we used an example where the user had to press the Return/Enter key to call the AddTwoNumbers()
method:
if(Input.GetKeyUp(Keycode.Return)) AddTwoNumbers();
The if
statement's condition becomes true only when the Return key is released after being pressed down.
Note
Notice that the code of AddTwoNumbers()
isn't between two curly braces. When there is only one line of code to execute for an if
or else
statement, you have the option of not using the curly braces.
Here's a partial screenshot of the GetKeyUp()
method as shown in Unity's Scripting Re ference:
