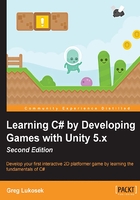
What is a method?
When we write a script, we are making lines of code that the computer is going to execute, one line at a time. As we write our code, there will be things that we want our game to execute more than once. For example, we can write a piece of code that adds two numbers. Suppose our game needs to add those two numbers a hundred different times during gameplay. So you'd say, "Wow! I have to write the same code a hundred times to add two numbers together? There has to be a better way."
Let a method take away your typing pain. You just have to write the code to add two numbers once and then give this chunk of code a name, such as AddTwoNumbers()
. Now, every time your game needs to add two numbers, don't write the code over and over; just call the AddTwoNumbers()
method.
Using the term "method" instead of "function"
You are constantly going to see the words "function" and "method" used everywhere as you learn how to code.
Note
The words "function" and "method" truly mean the same thing in Unity. They also do the same thing.
Since you are studying C#, and C# is an Object-Oriented Programming (OOP) language, I will use the word method throughout this book, just to be consistent with C# guidelines. It makes sense to learn the correct terminology for C#. The authors of Scripting Reference probably should have used the word "method" instead of "function" in all of their documentation. Anyway! Whenever you hear either of these words, remember that they both mean the same thing.
Note
From now on, I'm going to use the word "method" or "methods" in this book. When I refer to the functions shown in Scripting Reference, I'm going to use the word "method" instead, just to be consistent throughout this book.
We're going to edit LearningScript
again. In the following screenshot, there are a few lines of code that look strange. We are not going to get into the details of what they mean in this chapter. We will discuss that in Chapter 4, Getting into the Details of Methods. Right now, I am just showing you a method's basic structure and how it works:
- In MonoDevelop, select
LearningScript
for editing. - Edit the file so that it looks exactly like what is shown in the following screenshot:
- Save the file.
In the previous screenshot, lines 6 and 7 will look familiar to you. They are variables, just as you learned in the previous section. There are two of them this time. These variables store the numbers that are going to be added.
Line 16 may look very strange to you. Don't concern yourself right now with how it works. Just know that it's a line of code that lets the script know when the Return/Enter key is pressed. On the keyboard method AddTwoNumbers
will be called into action.
Note
The simplest way to call a function in your code is by using its name followed by braces and a semicolon, for example, AddTwoNumbers();
.
Method names are substitutes, too
You learned that a variable is a substitute for the value that it actually contains. Well, a method is no different. Take a look at line 20 in the previous screenshot:
void AddTwoNumbers ()
AddTwoNumbers()
is the name of the method. Like a variable, AddTwoNumbers()
is nothing more than a named placeholder in the memory, but this time, it stores some lines of code instead. So, wherever we wish to use the code in this method in our script, we just write AddTwoNumbers()
and the code will be substituted.
Line 20 has an opening curly brace and line 23 has a closing curly brace. Everything between the two curly braces is the code that is executed when this method is called in our script. Look at line 16 from the previous screenshot, precisely at this part:
AddTwoNumbers();
The method named AddTwoNumbers()
is called. This means that the code between the curly braces is executed. Of course, this AddTwoNumbers()
method has only one line of code to execute, but a method can have many lines of code.
Line 22 is the action part of this method—the part between the curly braces. This line of code adds the two variables and displays the answer on the Unity Console.
Then, follow these steps:
- Go back to Unity and have the Console panel showing.
- Now click on Play.
Oh no! Nothing happened! Hold on… Actually, as you sit there looking at the blank Console panel, the script is running perfectly, just as we programmed it. The first part of line 16 in the script is waiting for you to press the Return/Enter key. Press it now.
And there you go! The following screenshot shows you the result of adding two variables that contain the numbers 2 and 9:

In our LearningScript line 16 waited for you to press the Return/Enter key. When you do this, AddTwoNumbers()
method, is executed. When you do this, line 17, which calls the AddTwoNumbers()
method, is executed. This allows the code block of the method, line 23, to add the values stored in the number1
and number2
variables.
While Unity is in the Play mode, select Main Camera so that its components appear in the Inspector panel. In the Inspector panel, locate LearningScript
and its two variables. Change the values, currently 2
and 9
, to something else. Make sure that you click on the Game panel so that it has focus. Then press the Return/Enter key again. You will see the result of the new addition in Console.
You just learned how a method works to allow a specific block of code to be called in order to perform a task. We didn't get into any of the wording details of methods here. This was just to show you fundamentally how they work. We'll get into the finer details of methods in a later chapter.