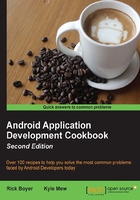
Changing layout properties during runtime
In Android development, it's generally the preferred practice to define the UI with XML and the application code in Java, keeping the User Interface code separate from the application code. There are times where it is much easier or more efficient, to alter (or even build) the UI from the Java code. Fortunately, this is easily supported in Android.
We saw a small example of modifying the layout from code in the previous recipe, where we set the number of GridView
column to display in the code. In this recipe, we will obtain a reference to the LayoutParams
object to change the margin during runtime.
Getting ready
Here we will set up a simple layout with XML and use a LinearLayout.LayoutParams
object to change the margins of a View during runtime.
How to do it....
- Open the
activity_main.xml
file and change the layout fromRelativeLayout
toLinearLayout
. It will look as follows:<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent"> </LinearLayout>
- Add a TextView and include an ID as follows:
android:id="@+id/textView"
- Add
Button
and include an ID as follows:android:id="@+id/button"
- Open
MainActivity.java
and add the following code to theonCreate()
method to set up anonClick
event listener:Button button = (Button)findViewById(R.id.button); button.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { ((TextView)findViewById(R.id.textView)).setText("Changed at runtime!"); LinearLayout.LayoutParams params = (LinearLayout.LayoutParams)view.getLayoutParams(); params.leftMargin += 5; } });
- Run the program on a device or emulator.
How it works...
Every View (and therefore ViewGroup
) has a set of layout parameters associated with it. In particular, all Views have parameters to inform their parent of their desired height and width. These are defined with the layout_height
and layout_width
parameters. We can access this layout information from the code with the getLayoutParams()
method. The layout information includes the layout height, width, margins, and any class-specific parameters. In this example, we moved the button on each click by obtaining the button LayoutParams
and changing the margin.