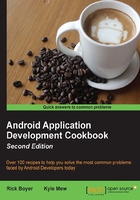
Using LinearLayout
Another common layout option is the LinearLayout
, which arranges the child Views in a single column or single row, depending on the orientation specified. The default orientation (if not specified) is vertical, which aligns the Views in a single column.
The LinearLayout
has a key feature not offered in the RelativeLayout
—the weight
attribute. We can specify a layout_weight
parameter when defining a View to allow the View to dynamically size based on the available space. Options include having a View fill all the remaining space (if a View has a higher weight), having multiple Views fit within the given space (if all have the same weight), or spacing the Views proportionally by their weight.
We will create a LinearLayout
with three EditText
Views to demonstrate how the weight attribute can be used. For this example, we will use three EditText
Views—one to enter a To Address
parameter, another to enter a Subject
, and the third to enter a Message
. The To
and Subject
Views will be a single line each, with the remaining space given to the Message View.
Getting ready
Create a new project and call it LinearLayout
. We will replace the default RelativeLayout
created in activity_main.xml
with a LinearLayout
.
How to do it...
- Open the
res/layout/activity_main.xml
file and replace it as follows:<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> <EditText android:id="@+id/editTextTo" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="To" /> <EditText android:id="@+id/editTextSubject" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="Subject" /> <EditText android:id="@+id/editTextMessage" android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="1" android:gravity="top" android:hint="Message" /> </LinearLayout>
- Run the code, or view the layout in the Design tab.
How it works...
When using vertical orientation with the LinearLayout
, the child Views are created in a single column (stacked on top of each other). The first two Views use the android:layout_height="wrap_content"
attribute, giving them a single line each. editTextMessage
uses the following to specify the height:
android:layout_height="0dp" android:layout_weight="1"
When using the LinearLayout
, it tells Android to calculate the height based on the weight. A weight of 0 (the default if not specified) indicates the View should not expand. In this example, editTextMessage
is the only View defined with a weight, so it alone will expand to fill any remaining space in the parent layout.
Tip
When using the horizontal orientation, specify android:layout_height="0dp"
(along with the weight) to have Android calculate the width.
It might be helpful to think of the weight attribute as a percentage. In this case, the total weight defined is 1, so this View gets 100 percent of the remaining space. If we assigned a weight of 1 to another View, the total would be 2, so this View would get 50 percent of the space. Try adding a weight to one of the other Views (make sure to change the height to 0dp
as well) to see it in action.
If you added a weight to one (or both) of the other Views, did you notice the text position? Without specifying a value for gravity
, the text just remains in the center of the View space. The editTextMessage
specifies: android:gravity="top"
, which forces the text to the top of the View.
There's more...
Multiple attribute options can be combined using bitwise OR
. (Java uses the pipe character (|) for OR
). For example, we could combine two gravity options to both align along the top of the parent and center within the available space:
android:layout_gravity="top|center"
It should be noted that the layout_gravity
and gravity
tags are not the same thing. Where layout_gravity
dictates where in its parent a View should lie, gravity
controls the positioning of the contents within a View—for example, the alignment of text on a button.
See also
- The previous recipe, Using the RelativeLayout