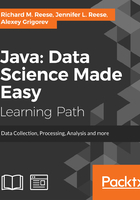
Validating data types
Sometimes we simply need to validate whether a piece of data is of a specific type, such as integer or floating point data. We will demonstrate in the next example how to validate integer data using the validateInt method. This technique is easily modified for the other major data types supported in the standard Java library, including Float and Double.
We need to use a try-catch block here to catch a NumberFormatException. If an exception is thrown, we know our data is not a valid integer. We first pass our text to be tested to the parseInt method of the Integer class. If the text can be parsed as an integer, we simply print out the integer. If an exception is thrown, we display information to that effect:
public static void validateInt(String toValidate){
try{
int validInt = Integer.parseInt(toValidate);
out.println(validInt + " is a valid integer");
}catch(NumberFormatException e){
out.println(toValidate + " is not a valid integer");
}
We will use the following method calls to test our method:
validateInt("1234");
validateInt("Ishmael");
The output follows:
1234 is a valid integer
Ishmael is not a valid integer
The Apache Commons contain an IntegerValidator class with additional useful functionalities. In this first example, we simply duplicate the process from before, but use IntegerValidator methods to accomplish our goal:
public static String validateInt(String text){
IntegerValidator intValidator = IntegerValidator.getInstance();
if(intValidator.isValid(text)){
return text + " is a valid integer";
}else{
return text + " is not a valid integer";
}
}
We again use the following method calls to test our method:
validateInt("1234");
validateInt("Ishmael");
The output follows:
1234 is a valid integer
Ishmael is not a valid integer
The IntegerValidator class also provides methods to determine whether an integer is greater than or less than a specific value, compare the number to a ranger of numbers, and convert Number objects to Integer objects. Apache Commons has a number of other validator classes. We will examine a few more in the rest of this section.