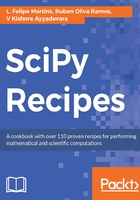
Creating an array with the same shape as another array
NumPy provides a family of functions that create an array with the same shape as another input array. These functions all end with the _like suffix, and are listed in the following table:

For example, the following code creates an array of zeros with the same shape as the x input array:
x = np.array([[1,2],[3,4],[5,6]], dtype=np.float64)
y = np.zeros_like(x)
This code first defines a 3 x 2 array, x. Then, it creates an array of zeros with the same shape as x, resulting in a 3 x 2 array of zeros.
It is important to notice that the type of the array constructed by the _like function is the same as the input array. So, the following code produces a slightly unexpected result:
x = np.array([[1,1],[2,2]])
z = np.full_like(x, 6.2)
The preceding code creates the following array:
array([[6, 6],
[6, 6]])
The programmer writing the previous code probably wanted to generate an array with the value 6.2 repeated in all positions. However, the array x in this example has a dtype of int64, so the full_like function will coerce the result to an array of 64-bit integers. To obtain the desired results, we can specify the type of array we want as follows:
z = np.full_like(x, 6.2, dtype=np.float64)
When in doubt, it is advisable to specify the data type of an array explicitly using the dtype option. As a matter of fact, I prefer to always specify the dtype of an array, to make my intentions clear.