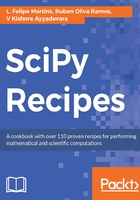
Selecting subarrays using an index list
A slice allows for the selection of items from an array from equally spaced rows or columns. If a more general subarray is required, we can use lists as indices. This is illustrated with the following code:
y = x[[2, 3, 1], [0, 3, 5]]
This will assign array([20, 33, 15]) to the array y. The code behaves as if the lists [2, 3, 1] and [0,3,5] are zipped into the sequence of indices (2,0), (3,3), and (1,5). Also notice that the resulting array y is one-dimensional.
Combined with slices, list indexes provides a powerful way to extract items from an array. The following example demonstrates how to select a subset of the columns of an array:
y = x[:, [5, 2, -1]]
dumpArray(y)
This code has the effect of selecting columns with indices 5 and 2, as well as the last column from the array x, and produces the following output:
05 02 09
15 12 19
25 22 29
...
Notice that when using a list array this way, we can reorder the rows/columns of an array.
As a final example, let's look at how to extract an arbitrary (rectangular) subarray. This can be done with the following code:
y = x[[2, 0, 3],:][:,[-2, 1]]
dumpArray(y)
This produces the following output:
28 21
08 01
38 31
The preceding code selects the subarray in two steps:
- First, the [[2, 0, 3],:] index expression selects rows 2, 0, and 3 from the array x.
- Then, [:,[-2, 1]] selects columns -2 and 1 from the array. Notice that -2 refers to the second column from the end of the array.