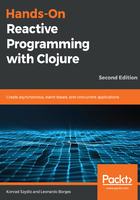
Higher-order FRP
Higher-order FRP refers to the original research on FRP that was developed by Conal Elliott and Paul Hudak in their paper Functional Reactive Animation[2] from 1997. This paper presents Fran, a domain-specific language embedded in Haskell for creating reactive animations. It has since been implemented in several languages as a library as well as purpose-built reactive languages.
If you recall the calculator example we created a few pages ago, we can see how that style of Reactive Programming requires us to manage state explicitly by directly reading and writing from/to the input fields. As Clojure developers, we know that avoiding state and mutable data is a good principle to keep in mind when building software. This principle is at the core of Functional Programming:
(->> [1 2 3 4 5 6] (map inc) (filter even?) (reduce +)) ;; 12
This short program increments all of the elements in the original list by one filters all even numbers, and adds them up using reduce.
Different from imperative programming, we focus on what we want to do, for example, iteration, and not how we want it to be done, for example, using a for loop. This is why the implementation matches our description of the program closely. This is known as declarative programming.
FRP brings the same philosophy to Reactive Programming. As the Haskell programming language wiki on the subject has wisely put it,
Put another way, FRP is a declarative way of modeling systems that respond to input over time.
Both statements touch on the concept of time. We'll be exploring that in the next section, where we introduce the key abstractions provided by FRP: signals (or behaviors) and events.