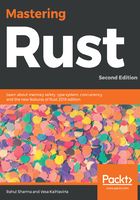
Declaring variables and immutability
Variables allow us to store a value and easily refer to it later in code. In Rust, we use the let keyword to declare variables. We already had a glimpse of it in the greet.rs example in the previous section. In mainstream imperative languages such as C or Python, initializing a variable does not stop you from reassigning it to some other value. Rust deviates from the mainstream here by making variables immutable by default, that is, you cannot assign the variable to some other value after you have initialized it. If you need a variable to point to something else (of the same type) later, you need to put the mut keyword before it. Rust asks you to be explicit about your intent as much as possible. Consider the following code:
// variables.rs
fn main() {
let target = "world";
let mut greeting = "Hello";
println!("{}, {}", greeting, target);
greeting = "How are you doing";
target = "mate";
println!("{}, {}", greeting, target);
}
We declared two variables, target and greeting. target is an immutable binding, while greeting has a mut before it, which makes it a mutable binding. If we run this program, though, we get the following error:

As you can see from the preceding error message, Rust does not let you assign to target again. To make this program compile, we'll need to add mut before target in the let statement and compile and run it again. The following is the output when you run the program:
$ rustc variables.rs
$ ./variables
Hello, world
How are you doing, mate
let does much more than assign variables. It is a pattern-matching statement in Rust. In Chapter 7, Advanced Concepts, we'll take a closer look at let. Next, we'll look at functions.